Invalid memory address or nil pointer dereference
yourbasic.org/golang
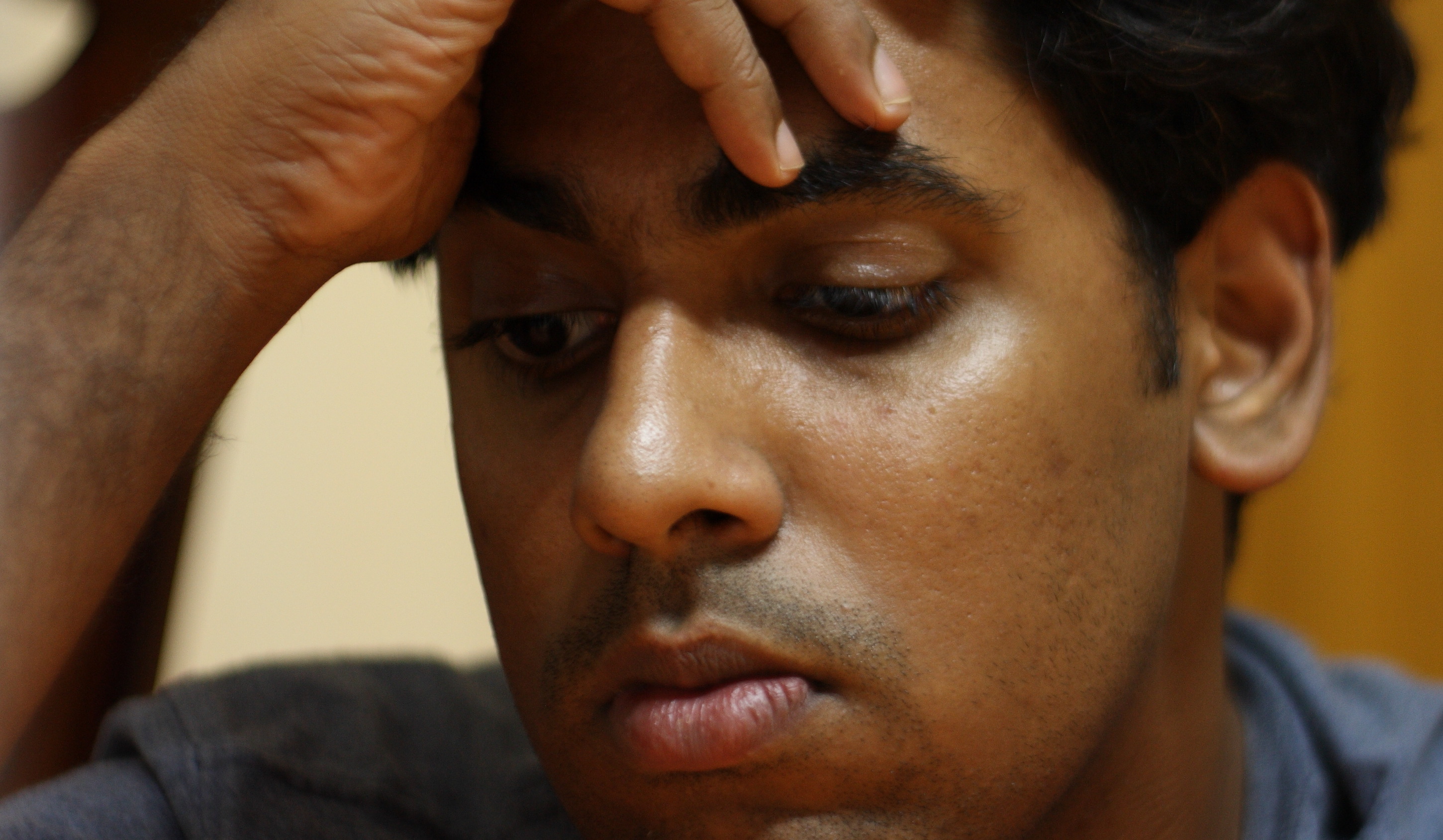
Why does this program panic?
type Point struct {
X, Y float64
}
func (p *Point) Abs() float64 {
return math.Sqrt(p.X*p.X + p.Y*p.Y)
}
func main() {
var p *Point
fmt.Println(p.Abs())
}
panic: runtime error: invalid memory address or nil pointer dereference
[signal SIGSEGV: segmentation violation code=0xffffffff addr=0x0 pc=0xd2c5a]
goroutine 1 [running]:
main.(*Point).Abs(...)
../main.go:6
main.main()
../main.go:11 +0x1a
Answer
The uninitialized pointer p
in the main
function is nil
,
and you can’t follow the nil pointer.
Ifx
is nil, an attempt to evaluate*x
will cause a run-time panic. The Go Programming Language Specification: Address operators
You need to create a Point
:
func main() {
var p *Point = new(Point)
fmt.Println(p.Abs())
}
Since methods with pointer receivers take either a value or a pointer, you could also skip the pointer altogether:
func main() {
var p Point // has zero value Point{X:0, Y:0}
fmt.Println(p.Abs())
}
See Pointers for more about pointers in Go.