Create a temporary file or directory
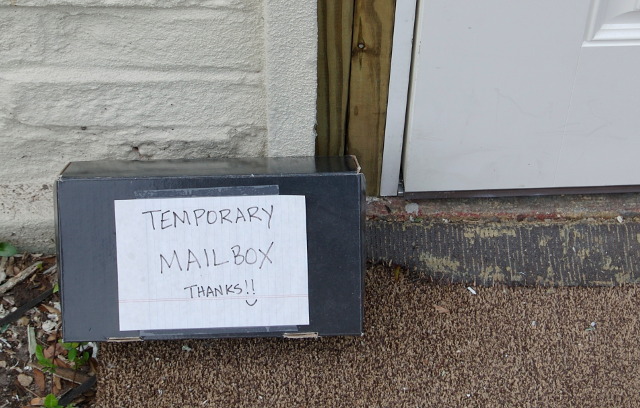
File
Use ioutil.TempFile
in package
io/ioutil
to create a
globally unique temporary file.
It’s your own job to remove the file when it’s no longer needed.
file, err := ioutil.TempFile("dir", "prefix")
if err != nil {
log.Fatal(err)
}
defer os.Remove(file.Name())
fmt.Println(file.Name()) // For example "dir/prefix054003078"
The call to ioutil.TempFile
- creates a new file with a name starting with
"prefix"
in the directory"dir"
, - opens the file for reading and writing,
- and returns the new
*os.File
.
To put the new file in os.TempDir()
,
the default directory for temporary files,
call ioutil.TempFile
with an empty directory string.
Add a suffix to the temporary file nameGo 1.11
Starting with Go 1.11, if the second string given to TempFile
includes a "*"
,
the random string replaces this "*"
.
file, err := ioutil.TempFile("dir", "myname.*.bat")
if err != nil {
log.Fatal(err)
}
defer os.Remove(file.Name())
fmt.Println(file.Name()) // For example "dir/myname.054003078.bat"
If no "*"
is included the old behavior is retained,
and the random digits are appended to the end.
Directory
Use ioutil.TempDir
in package
io/ioutil
to create a
globally unique temporary directory.
dir, err := ioutil.TempDir("dir", "prefix")
if err != nil {
log.Fatal(err)
}
defer os.RemoveAll(dir)
The call to ioutil.TempDir
- creates a new directory with a name starting with
"prefix"
in the directory"dir"
- and returns the path of the new directory.
To put the new directory in os.TempDir()
,
the default directory for temporary files,
call ioutil.TempDir
with an empty directory string.