Print null in Java
What happens if you try to print null in Java? It depends.
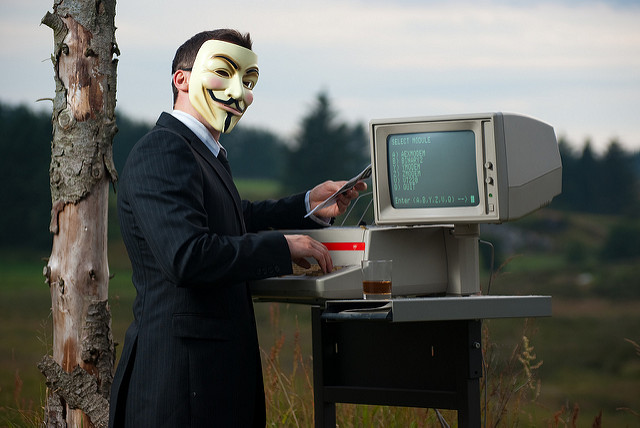
What the compiler said
The following line will not compile.
System.out.println(null);
This is the message from my compiler.
reference to println is ambiguous, both method println(char[]) in java.io.PrintStream and method println(java.lang.String) in java.io.PrintStream match
In fact, println(java.lang.Object)
in java.io.PrintStream
is yet another match,
but Java has a way of choosing between that one and each of the two methods above.
It’s just the string and the character array parameters
that cause ambiguity; character arrays and objects can happily coexist.
I’m not making this up.
What the runtime did
The following lines will compile.
Object o = null; String s = null; System.out.println(o); System.out.println(s);
Here is the output.
null null
The following will also compile.
char[] a = null; System.out.println(a);
But this method throws an exception.
Exception in thread "main" java.lang.NullPointerException at java.io.Writer.write(Writer.java:127) at java.io.PrintStream.write(PrintStream.java:470) at java.io.PrintStream.print(PrintStream.java:620) at java.io.PrintStream.println(PrintStream.java:759) ...
With some more research funding I might be able to figure this out!
Key takeaways
Method overloading and inheritance are two fairly complicated constructs. If you combine them, things can get out of control. And this can happen when you least expect it.
Here are two rules of thumb from Effective Java that can help.
A safe, conservative policy is never to export two overloadings with the same number of parameters. (Item 41)
Use composition and forwarding instead of inheritance, especially if an appropriate interface to implement a wrapper class exists. (Item 16)
Further reading
Go vs. Java: 15 main differences is a brief code comparison.
This Java to Go in-depth tutorial is intended to help Java developers come up to speed quickly with Go.