What’s a seed in a random number generator?
In reality pseudorandom numbers aren't random at all. They are computed using a fixed deterministic algorithm.
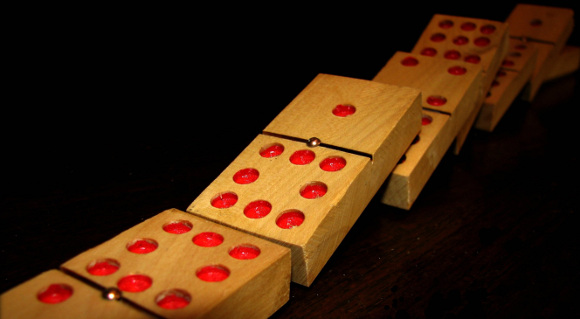
The seed is a starting point for a sequence of pseudorandom numbers. If you start from the same seed, you get the very same sequence. This can be quite useful for debugging.
If you want a different sequence of numbers each time, you can use the current time as a seed.
Example
This generator produces a sequence of 97 different numbers, then it starts over again. The seed decides at what number the sequence will start.
// New returns a pseudorandom number generator Rand with a given seed.
// Every time you call Rand, you get a new "random" number.
func New(seed int) (Rand func() int) {
current := seed
return func() int {
next := (17 * current) % 97
current = next
return next
}
}
func main() {
rand1 := New(1)
fmt.Println(rand1(), rand1(), rand1())
rand2 := New(2)
fmt.Println(rand2(), rand2(), rand2())
}
17 95 63 34 93 29
The random number generators you’ll find in most programming languages
work just like this, but of course they use a smarter function.
Ideally, you want a long sequence with good random properties
computed by a function which uses only cheap arithmetic operations.
For example, you would typically want to avoid the %
modulus operator.