Go step by step
yourbasic.org/golang
Detailed descriptions of core Go concepts
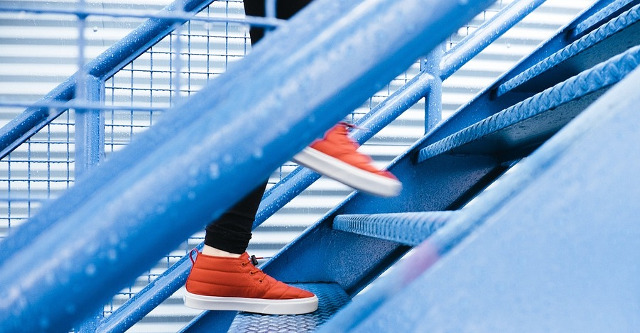
Table of contents
-
Type, value and equality of interfaces
An interface type consists of a set of method signatures. A variable of interface type can hold any value that implements these methods.
-
Create, initialize and compare structs
A struct is a typed collection of fields, useful for grouping data into records.
-
Slices/arrays explained: create, index, slice, iterate
A slice refers to a section of an underlying array. It can grow and shrink within the bounds of this array.
-
Maps explained: create, add, get, delete
Go maps are implemented by hash tables and offer efficient add, get and delete operations. You create a new map with a make statement or a map literal.
-
5 basic for loop patterns
The for loop can be used for three-component loops, while loops, infinite loops and for-each range loops. You can exit a loop with break or continue.
-
5 switch statement patterns
A switch-case-default statement is a shorter and cleaner way to write a sequence of if-else statements.
-
Packages explained: declare, import, download, document
Every Go program is made up of packages and each package has an import path.
Further reading
This Concurrent programming tutorial covers the fundamentals of concurrent programming with examples in Go.