How to kill a goroutine
yourbasic.org/golang
One goroutine can't forcibly stop another.
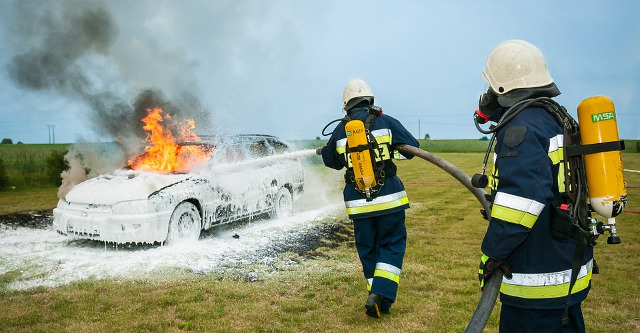
To make a goroutine stoppable, let it listen for a stop signal on a dedicated quit channel, and check this channel at suitable points in your goroutine.
quit := make(chan bool)
go func() {
for {
select {
case <-quit:
return
default:
// …
}
}
}()
// …
quit <- true
Here is a more complete example, where we use a single channel for both data and signalling.
// Generator returns a channel that produces the numbers 1, 2, 3,…
// To stop the underlying goroutine, send a number on this channel.
func Generator() chan int {
ch := make(chan int)
go func() {
n := 1
for {
select {
case ch <- n:
n++
case <-ch:
return
}
}
}()
return ch
}
func main() {
number := Generator()
fmt.Println(<-number)
fmt.Println(<-number)
number <- 0 // stops underlying goroutine
fmt.Println(<-number) // error, no one is sending anymore
// …
}
1
2
fatal error: all goroutines are asleep - deadlock!