Concurrent programming
yourbasic.org/golang
This tutorial covers the fundamentals of concurrent programming with examples in Go.
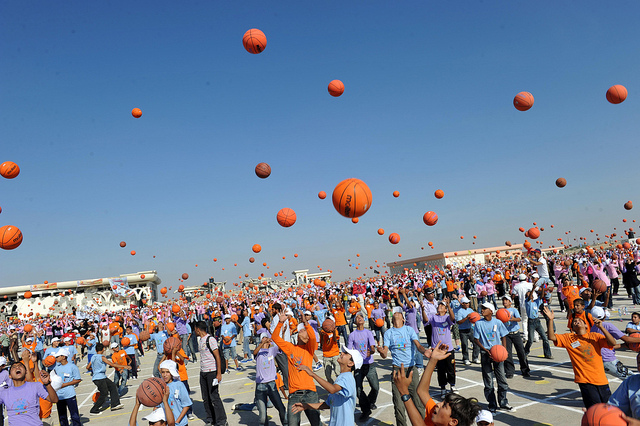
Before you start, you need to know how to write basic Go programs. If you need a refresher, the resources in this Go beginner’s guide will help you come up to speed quickly with Go.
Table of contents
-
Goroutines are lightweight threads
A goroutine is a lightweight thread of execution. All goroutines in a single program share the same address space.
-
Channels offer synchronized communication
A channel is a mechanism for two goroutines to synchronize execution and communicate by passing values.
-
Select waits on a group of channels
A select statement allows you to wait for multiple send or receive operations simultaneously.
-
Data races explained
A data race is easily introduced by mistake and can lead to situations that are very hard to debug. This article explains how to avoid this headache.
-
How to detect data races
By starting your application with the '-race' option, the Go runtime might be able to detect and inform you about data races.
-
How to debug deadlocks
The Go runtime can often detect when a program freezes because of a deadlock. This article explains how to debug and solve such issues.
-
Waiting for goroutines
A sync.WaitGroup waits for a group of goroutines to finish.
-
Broadcast a signal on a channel
When you read from a closed channel, you receive a zero value. This can be used to broadcast a signal to several goroutines on a single channel.
-
How to kill a goroutine
One goroutine can't forcibly stop another. To make a goroutine stoppable, let it listen for a stop signal on a channel.
-
Timer and Ticker: events in the future
Timers and Tickers are used to wait for, repeat, and cancel events in the future.
-
Mutual exclusion lock (mutex)
A sync.Mutex is used to synchronize data by explicit locking in Go.
-
3 rules for efficient parallel computation
To efficiently schedule parallel computation on separate CPUs is more of an art than a science. This article gives some rules of thumb.
More Go tutorials
Tutorials for beginners, best practices, cheat sheets and production-quality code examples.