How to use the io.Writer interface
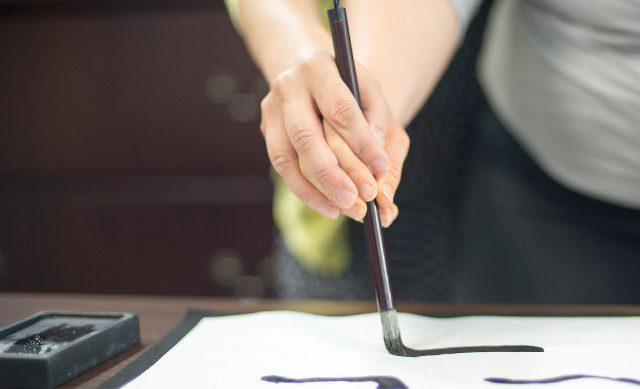
Basics
The io.Writer
interface represents an entity
to which you can write a stream of bytes.
type Writer interface {
Write(p []byte) (n int, err error)
}
Write
writes up to len(p)
bytes from p
to the underlying data stream –
it returns the number of bytes written and any error encountered that caused
the write to stop early.
The standard library provides numerous Writer implementations, and Writers are accepted as input by many utilities.
How to use a built-in writer (3 examples)
As a first example, you can write directly into a bytes.Buffer
using the fmt.Fprintf
function.
This works since
bytes.Buffer
has aWrite
method, andfmt.Fprintf
takes aWriter
as its first argument.
var buf bytes.Buffer
fmt.Fprintf(&buf, "Size: %d MB.", 85)
s := buf.String()) // s == "Size: 85 MB."
Similarly, you can write directly into files or other streams, such as http connections. See the HTTP server example article for a complete code example.
This is a very common pattern in Go.
As yet another example, you can compute the hash value of a file
by copying the file into the io.Writer
function
of a suitable hash.Hash
object.
See Hash checksums
for code.
Optimize string writes
Some Writers in the standard library have an additional WriteString
method.
This method can be more efficient than the standard Write
method
since it writes a string directly without allocating a byte slice.
You can take direct advantage of this optimization by using the
io.WriteString()
function.
func WriteString(w Writer, s string) (n int, err error)
If w
implements a WriteString
method, it is invoked directly.
Otherwise, w.Write
is called exactly once.