Why Go? – Key advantages you may have overlooked
Go makes it easier (than Java or Python) to write correct, clear and efficient code.
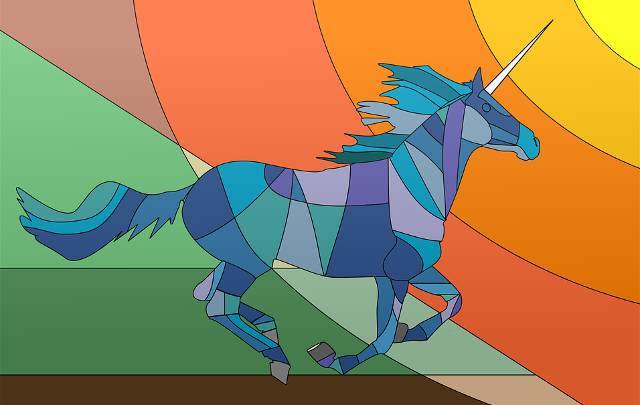
Choosing a programming language isn’t easy. The separate features of a language may look great at first, but it takes time and experience to spot the drawbacks.
As a CS professor and longtime Go and Java developer, I’d like to share some of my thoughts and explain why I prefer Go to Java or Python – Go makes it much easier for me to write good code.
Go has been my main programming tool since 2012, replacing Java, which in turn replaced C in 1998. I use Python mostly for teaching. My journey as a programmer started back in 1978 on a TI-57 with 50 program steps and 8 registers.
Minimalism
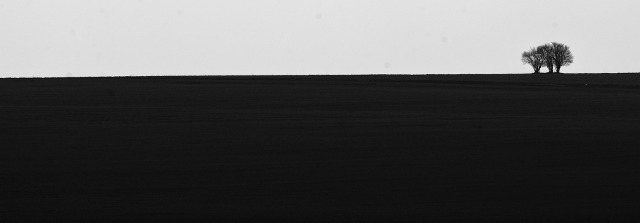
Go is a minimalist language, and that’s (mostly) a blessing.
The formal Go language specification is only 50 pages, has plenty of examples, and is fairly easy to read. A skilled programmer could probably learn Go from the specification alone.
The core language consists of a few simple, orthogonal features that can be combined in a relatively small number of ways. This makes it easier to learn the language, and to read and write programs.
When you add new features to a language, the complexity doesn’t just add up, it often multiplies: language features can interact in many ways. This is a significant problem – language complexity affects all developers (not just the ones writing the spec and implementing the compiler).
Here are some core Go features:
- The built-in frameworks for testing and profiling are small and easy to learn, but still fully functional. There are plenty of third-party add-ons, but chances are you won’t need them.
- It’s possible to debug and profile an optimized binary running in production through an HTTP server.
- Go has automatically generated documentation with testable examples. Once again, the interface is minimal, and there is very little to learn.
- Go is strongly and statically typed with no implicit conversions, but the syntactic overhead is still surprisingly small. This is achieved by simple type inference in assignments together with untyped numeric constants. This gives Go stronger type safety than Java (which has implicit conversions), but the code reads more like Python (which has untyped variables).
- Programs are constructed from packages that offer clear code separation and allow efficient management of dependencies. The package mechanism is perhaps the single most well-designed feature of the language, and certainly one of the most overlooked.
- Structurally typed interfaces provide runtime polymorphism through dynamic dispatch.
- Concurrency is an integral part of Go, supported by goroutines, channels and the select statement.
See Go vs. Java: 15 main differences for a small code example, and a sample of basic data types, methods and control structures.
Features for the future
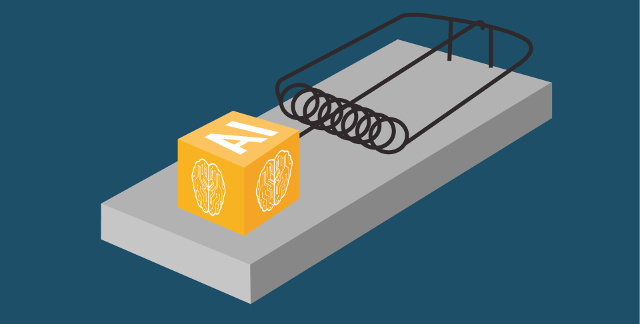
Go omits several features found in other modern languages.
Here’s the language designers' answer to Why does Go not have feature X?
Every language contains novel features and omits someone’s favorite feature. Go was designed with an eye on felicity of programming, speed of compilation, orthogonality of concepts, and the need to support features such as concurrency and garbage collection. Your favorite feature may be missing because it doesn’t fit, because it affects compilation speed or clarity of design, or because it would make the fundamental system model too difficult.
New features are considered only if there is a pressing need demonstrated by experience reports from real-world projects.
There are a few likely major additions in the pipeline:
- Package management through modules were preliminary introduced in Go 1.11.
- There is a generics draft design, which may be implemented in Go 2. For now, have a look at Generics (alternatives and workarounds).
- Similarly, there is an error handling draft design that extends the current minimalist error handling.
Currently, some minor additions are considered to help create and test a community-driven process for developing Go.
However, features such as optional parameters, default parameter values and method overloading probably won’t be part of a future Go 2.
Java comparison
The Java® Language Specification is currently 750 pages. Much of the complexity is due to feature creep. Here are but three examples.
Java inner classes suddenly appeared in 1997; it took more than a year to update the specification, and it became almost twice as big as a result. That’s a high price to pay for a non-essential feature.
Generics in Java, implemented by type erasure, make some code cleaner and allow for additional runtime checks, but quickly become complex when you move beyond basic examples: generic arrays aren’t supported and type wildcards with upper and lower bounds are quite complicated. The string “generic” appears 280 times in the specification. It’s not clear to me if this feature is worth its cost.
A Java enum, introduced in 2004, is a special type of class that represents a group of constants. It’s certainly nice to have, but offers little that couldn’t be done with ordinary classes. The string “enum” appears 241 times in the specification.
Code transparency
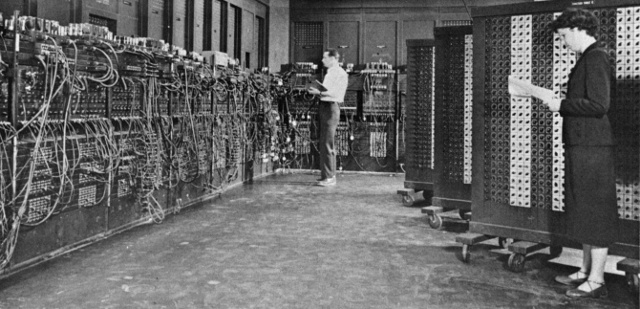
Your project is doomed if you can’t understand your code.
- You always need to know exactly what your coding is doing,
- and sometimes need to estimate the resources (time and memory) it uses.
Go tries to meet both of these goals.
The syntax is designed to be transparent and there is one standard code format, automatically generated by the fmt tool.
Another example is that Go programs with unused package imports do not compile. This improves code clarity and long-term performance.
I suspect that the Go designers made some things difficult on purpose. You need to jump through hoops to catch a panic (exception) and you are forced to sprinkle your code with the word “unsafe” to step around type safety.
Python comparison
The Python code snippet del a[i]
deletes the element at index i
from a list a
.
This code certainly is quite readable, but not so transparent:
it’s easy to miss that the time complexity
is O(n), where n is the number of elements in the list.
Go doesn’t have a similar utility function. This is definitely less convenient, but also more transparent. Your code needs to explicitly state if it copies a section of the list. See 2 ways to delete an element from a slice for a Go code example.
Java comparison
Code transparency is not just a syntactic issue. Here are two examples where the rules for Go package initialization and program execution order make it easier to reason about and maintain a project.
-
Circular dependencies can cause many unwanted effects. As opposed to Java, a Go program with initialization cycles will not compile.
-
When the main function of a Go program returns, the program exits. A Java programs exits when all user non-daemon threads finish.
This means that you may need to study large parts of a Java program to understand some of its behavior. This may even be impossible if you use third-party libraries.
Compatibility
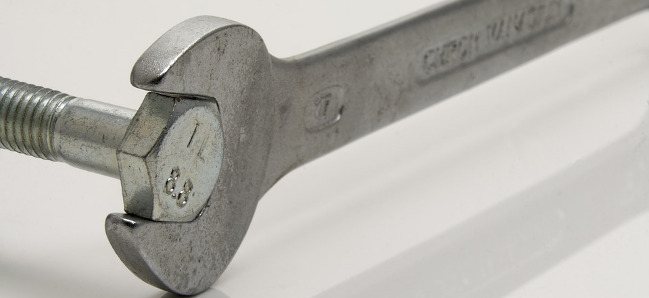
A language that changes abruptly, or becomes unavailable, can end your project.
Go 1 has succinct and strict compatibility guarantees for the core language and standard packages – Go programs that work today should continue to work with future releases of Go 1. Backward compatibility has been excellent so far.
Go is an open source project and comes with a BSD-style license that permits commercial use, modification, distribution, and private use. Copyright belongs to The Go Authors, those of us who contributed to the project. There is also a patent grant by Google.
Python comparison
If you’re a Python developer, you know the pain of having to deal with the differences between Python 2.7.x and Python 3.x. There are strong reasons for choosing Python 3, but if you depend on libraries that are only available for an older version, you may not be able.
Java comparison
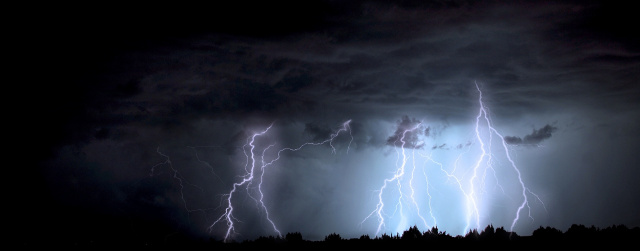
Java has a very good history of backward compatibility and the Compatibility Guide for JDK 8 is extensive. Also, Java has been freely available to developers for a long time.
Unfortunately, there are some dark clouds on the horizon with the Oracle America, Inc. v. Google, Inc. legal case about the nature of computer code and copyright law, and Oracle’s new Java licensing model.
Performance
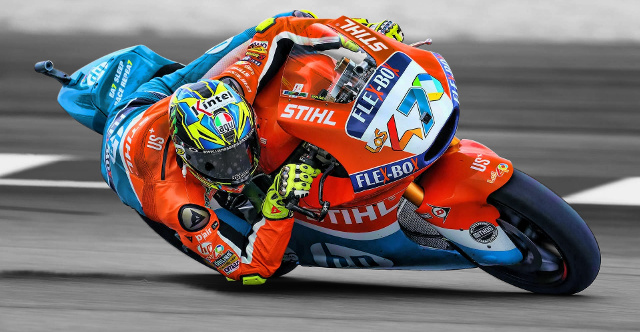
The exterior of Go is far from flashy, but there is a fine-tuned engine underneath.
It makes little sense to discuss performance issues out of context. Running time and memory use is heavily influenced by factors such as algorithms, data structures, input, coding skill, operating systems, and hardware.
Still, language, runtime and standard libraries can have a large effect on performance. This discussion is limited to high-level issues and design decisions. See the Go FAQ for a more detailed look at the implementation and its performance.
First, Go is a compiled language. An executable Go program typically consists of a single standalone binary, with no separate dynamic libraries or virtual machines, which can be directly deployed.
Size and speed of generated code will vary depending on target architecture. Go code generation is fairly mature and the major OSes (Linux, macOS, Windows) and architectures (Intel x86/x86-64, ARM64, WebAssembly, ARM), as well as many others, are supported. You can expect performance to be on a similar level to that of C++ or Java. Compared to interpreted Python code, the improvement can be huge.
Go is garbage collected, protecting against memory leaks. The collection has very low latency. In fact, you may never notice that the GC thread is there.
The standard libraries are typically of high quality, with optimized code using efficient algorithms. As an example, regular expressions are very efficient in Go with running time linear in the size of the input. Unfortunately, this is not true for Java and Python.
Build speeds, in absolute terms, are currently fairly good. More importantly, Go is designed to make compilation and dependency analysis easy, making it possible to create programming tools that scales well with growing projects.