Pointers explained
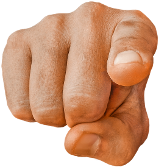
A pointer is a variable that contains the address of an object.
Basics
Structs and arrays are copied when used in assignments and passed as arguments to functions. With pointers this can be avoided.
Pointers store addresses of objects. The addresses can be passed around more efficiently than the actual objects.
A pointer has type *T
.
The keyword new
allocates a new object and returns its address.
type Student struct {
Name string
}
var ps *Student = new(Student) // ps holds the address of the new struct
The variable declaration can be written more compactly.
ps := new(Student)
Address operator
The &
operator returns the address of an object.
s := Student{"Alice"} // s holds the actual struct
ps := &s // ps holds the address of the struct
The &
operator can also be used with composite literals.
The two lines above can be written as
ps := &Student{"Alice"}
Pointer indirection
For a pointer x
, the pointer indirection *x
denotes the value which x
points to.
Pointer indirection is rarely used,
since Go can automatically take the address of a variable.
ps := new(Student)
ps.Name = "Alice" // same as (*ps).Name = "Alice"
Pointers as parameters
When using a pointer to modify an object, you’re affecting all code that uses the object.
// Bob is a function that has no effect.
func Bob(s Student) {
s.Name = "Bob" // changes only the local copy
}
// Charlie sets pp.Name to "Charlie".
func Charlie(ps *Student) {
ps.Name = "Charlie"
}
func main() {
s := Student{"Alice"}
Bob(s)
fmt.Println(s) // prints {Alice}
Charlie(&s)
fmt.Println(s) // prints {Charlie}
}