3 ways to split a string into a slice
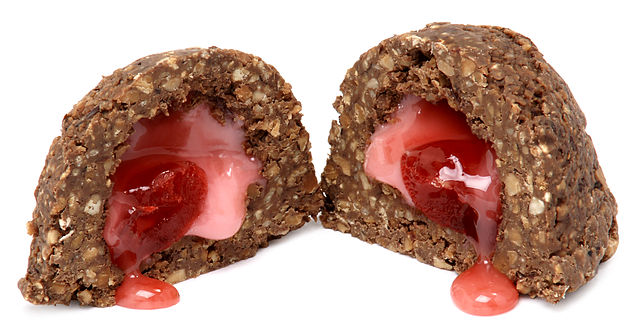
Split on comma or other substring
Use the strings.Split
function to split a string into its comma separated values.
s := strings.Split("a,b,c", ",")
fmt.Println(s)
// Output: [a b c]
To include the separators, use strings.SplitAfter
.
To split only the first n values, use strings.SplitN
and strings.SplitAfterN
.
You can use strings.TrimSpace
to strip leading and trailing whitespace from the resulting strings.
Split by whitespace and newline
Use the strings.Fields
function to split a string into substrings removing any space characters,
including newlines.
s := strings.Fields(" a \t b \n")
fmt.Println(s)
// Output: [a b]
Split on regular expression
In more complicated situations,
the regexp Split
method might do the trick.
It splits a string into substrings separated by a regular expression.
The method takes an integer argument n
;
if n >= 0
, it returns at most n
substrings.
a := regexp.MustCompile(`a`) // a single `a`
fmt.Printf("%q\n", a.Split("banana", -1)) // ["b" "n" "n" ""]
fmt.Printf("%q\n", a.Split("banana", 0)) // [] (nil slice)
fmt.Printf("%q\n", a.Split("banana", 1)) // ["banana"]
fmt.Printf("%q\n", a.Split("banana", 2)) // ["b" "nana"]
zp := regexp.MustCompile(` *, *`) // spaces and one comma
fmt.Printf("%q\n", zp.Split("a,b , c ", -1)) // ["a" "b" "c "]
See this Regexp tutorial and cheat sheet for a gentle introduction to the Go regexp package with plenty of examples.