Table-driven unit tests
yourbasic.org/golang
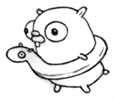
Here is the code we want to test.
package search
// Find returns the smallest index i at which x <= a[i].
// If there is no such index, it returns len(a).
// The slice must be sorted in ascending order.
func Find(a []int, x int) int {
switch len(a) {
case 0:
return 0
case 1:
if x <= a[0] {
return 0
}
return 1
}
mid := len(a) / 2
if x <= a[mid-1] {
return Find(a[:mid], x)
}
return mid + Find(a[mid:], x)
}
- Put the test code in a file whose name ends with _test.go.
- Write a function
TestXXX
with a single argument of type*testing.T
. The test framework runs each such function. - To indicate a failed test, call a failure function such as
t.Errorf
.
package search
import "testing"
var tests = []struct {
a []int
x int
exp int
}{
{[]int{}, 1, 0},
{[]int{1, 2, 3, 3}, 0, 0},
{[]int{1, 2, 3, 3}, 1, 0},
{[]int{1, 2, 3, 3}, 2, 1},
{[]int{1, 2, 3, 3}, 3, 3}, // incorrect test case
{[]int{1, 2, 3, 3}, 4, 4},
}
func TestFind(t *testing.T) {
for _, e := range tests {
res := Find(e.a, e.x)
if res != e.exp {
t.Errorf("Find(%v, %d) = %d, expected %d",
e.a, e.x, res, e.exp)
}
}
}
Run the tests with go test
.
$ go test --- FAIL: TestFind (0.00s) search_test.go:22: Find([1 2 3 3], 3) = 2, expected 3 FAIL exit status 1 FAIL .../search 0.001s
Further reading
The Induction and recursive functions article
has a correctness proof for the Find
function.
More code examples
Go blueprints: code for common tasks is a collection of handy code examples.