Untyped numeric constants with no limits
yourbasic.org/golang
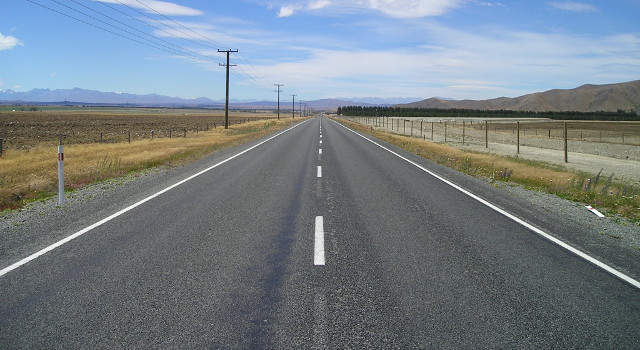
Constants may be typed or untyped.
const a uint = 17
const b = 55
An untyped constant has no limits. When it’s used in a context that requires a type, a type will be inferred and a limit applied.
const big = 10000000000 // Ok, even though it's too big for an int.
const bigger = big * 100 // Still ok.
var i int = big / 100 // No problem: the new result fits in an int.
// Compile time error: "constant 10000000000 overflows int"
var j int = big
The inferred type is determined by the syntax of the value:
123
gets typeint
, and123.4
becomes afloat64
.
The other possibilities are rune
(alias for int32
) and complex128
.
Enumerations
Go does not have enumerated types. Instead, you can use the special name iota
in a single const
declaration to get a series of increasing values. When an initialization expression is omitted for a const
, it reuses the preceding expression.
const (
red = iota // red == 0
blue // blue == 1
green // green == 2
)
See 4 iota enum examples for further examples.