Unused local variables
yourbasic.org/golang
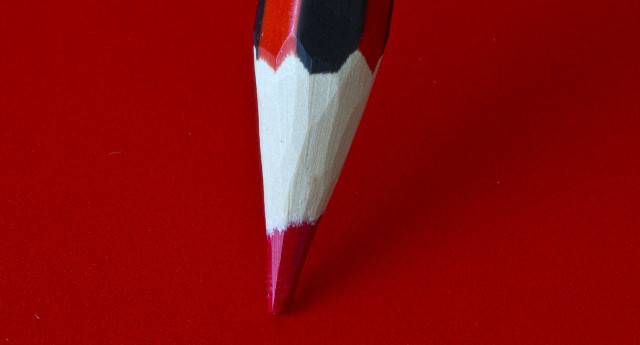
As you may have noticed, programs with unused local variables do not compile.
func main() {
var n int // "n declared and not used"
n = 5 // this doesn't help
}
../main.go:2:6: n declared and not used
This is a deliberate feature of the Go language.
The presence of an unused variable may indicate a bug […] Go refuses to compile programs with unused variables or imports, trading short-term convenience for long-term build speed and program clarity. Go FAQ: Can I stop these complaints about my unused variable/import?
Unused global variables and function arguments are however allowed.
Workaround
If you don’t want to remove or comment out the declaration, you can add a dummy assignment.
func main() {
var n int
n = 5
_ = n // n is now "used"
}
Further reading
Learn to love your compiler is a list of common Go compiler error messages: what they mean and how to fix the problem.