Access private fields with reflection
yourbasic.org/golang
With reflection it's possible to read, but not write, unexported fields of a struct defined in another package.
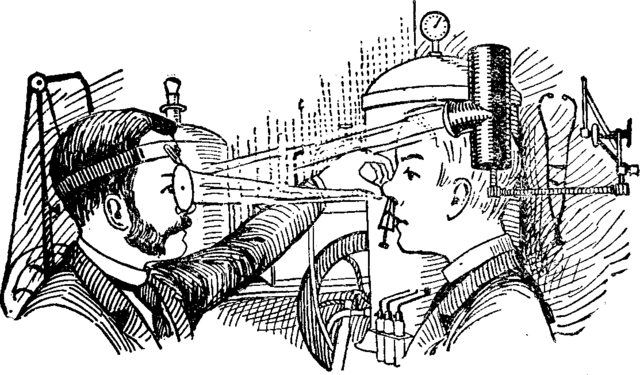
In this example, we access the unexported field len
in the
List
struct in package container/list
:
package list
type List struct {
root Element
len int
}
This code reads the value of len
with reflection.
package main
import (
"container/list"
"fmt"
"reflect"
)
func main() {
l := list.New()
l.PushFront("foo")
l.PushFront("bar")
// Get a reflect.Value fv for the unexported field len.
fv := reflect.ValueOf(l).Elem().FieldByName("len")
fmt.Println(fv.Int()) // 2
// Try to set the value of len.
fv.Set(reflect.ValueOf(3)) // ILLEGAL
}
2
panic: reflect: reflect.Value.Set using value obtained using unexported field
goroutine 1 [running]:
reflect.flag.mustBeAssignable(0x1a2, 0x285a)
/usr/local/go/src/reflect/value.go:225 +0x280
reflect.Value.Set(0xee2c0, 0x10444254, 0x1a2, 0xee2c0, 0x1280c0, 0x82)
/usr/local/go/src/reflect/value.go:1345 +0x40
main.main()
../main.go:18 +0x280
More code examples
Go blueprints: code for common tasks is a collection of handy code examples.