Find the type of an object
yourbasic.org/golang
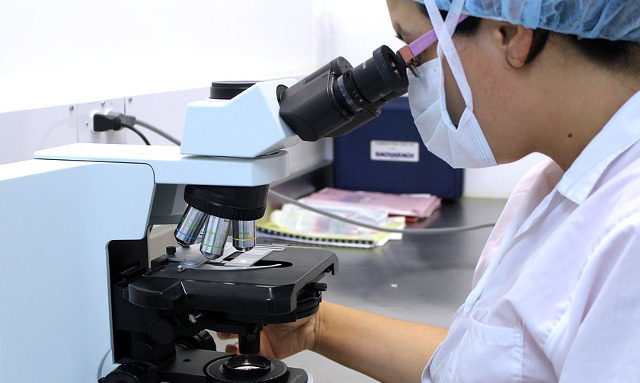
Use fmt for a string type description
You can use the %T
flag in the fmt package
to get a Go-syntax representation of the type.
var x interface{} = []int{1, 2, 3}
xType := fmt.Sprintf("%T", x)
fmt.Println(xType) // "[]int"
(The empty interface denoted by interface{}
can hold values of any type.)
A type switch lets you choose between types
Use a type switch to do several type assertions in series.
var x interface{} = 2.3
switch v := x.(type) {
case int:
fmt.Println("int:", v)
case float64:
fmt.Println("float64:", v)
default:
fmt.Println("unknown")
}
// Output: float64: 2.3
Reflection gives full type information
Use the reflect package if the options above don’t suffice.
var x interface{} = []int{1, 2, 3}
xType := reflect.TypeOf(x)
xValue := reflect.ValueOf(x)
fmt.Println(xType, xValue) // "[]int [1 2 3]"