Methods explained
yourbasic.org/golang
Go doesn't have classes, but you can define methods on types.
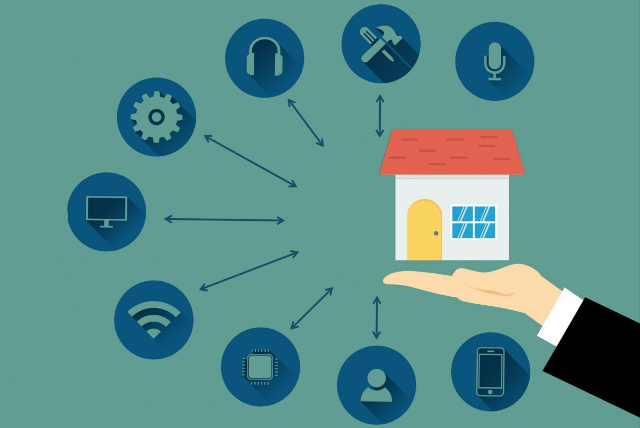
You can define methods on any type declared in a type definition.
- A method is a function with an extra receiver argument.
- The receiver sits between the
func
keyword and the method name.
In this example, the HasGarage
method is associated with the House
type.
The method receiver is called p
.
type House struct {
garage bool
}
func (p *House) HasGarage() bool { return p.garage }
func main() {
house := new(House)
fmt.Println(house.HasGarage()) // Prints "false" (zero value)
}
Conversions and methods
If you convert a value to a different type, the new value will have the methods of the new type, but not the old.
type MyInt int
func (m MyInt) Positive() bool { return m > 0 }
func main() {
var m MyInt = 2
m = m * m // The operators of the underlying type still apply.
fmt.Println(m.Positive()) // Prints "true"
fmt.Println(MyInt(3).Positive()) // Prints "true"
var n int
n = int(m) // The conversion is required.
n = m // ILLEGAL
}
../main.go:14:4: cannot use m (type MyInt) as type int in assignment
It’s idiomatic in Go to convert the type of an expression to access a specific method.
var n int64 = 12345
fmt.Println(n) // 12345
fmt.Println(time.Duration(n)) // 12.345µs
(The underlying type of time.Duration
is int64
,
and the time.Duration
type has a String
method
that returns the duration formatted as a time.)
Further reading
Object-oriented programming without inheritance explains how composition, embedding and interfaces support code reuse and polymorphism in Go.