Shuffle a slice or array
yourbasic.org/golang
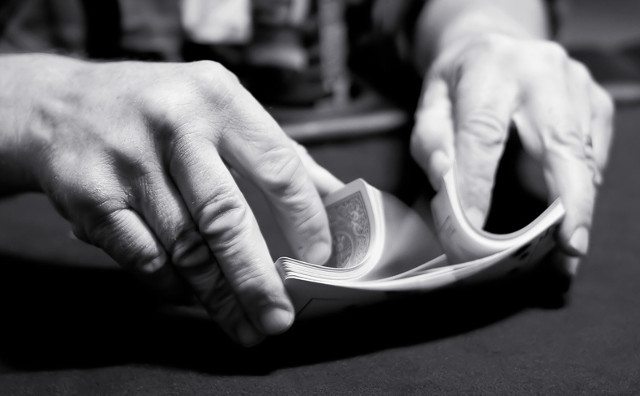
The rand.Shuffle
function
in package math/rand
shuffles an input sequence using a given swap function.
a := []int{1, 2, 3, 4, 5, 6, 7, 8}
rand.Seed(time.Now().UnixNano())
rand.Shuffle(len(a), func(i, j int) { a[i], a[j] = a[j], a[i] })
[5 8 6 4 3 7 2 1]
Warning: Without the call to rand.Seed
you will get
the same sequence of pseudorandom numbers each time you run the program.
Further reading
Generate random numbers, characters and slice elements
Before Go 1.10
Use the rand.Seed
and
rand.Intn
functions in package
math/rand
.
a := []int{1, 2, 3, 4, 5, 6, 7, 8}
rand.Seed(time.Now().UnixNano())
for i := len(a) - 1; i > 0; i-- { // Fisher–Yates shuffle
j := rand.Intn(i + 1)
a[i], a[j] = a[j], a[i]
}