Go blueprints: code for common tasks
yourbasic.org/golang
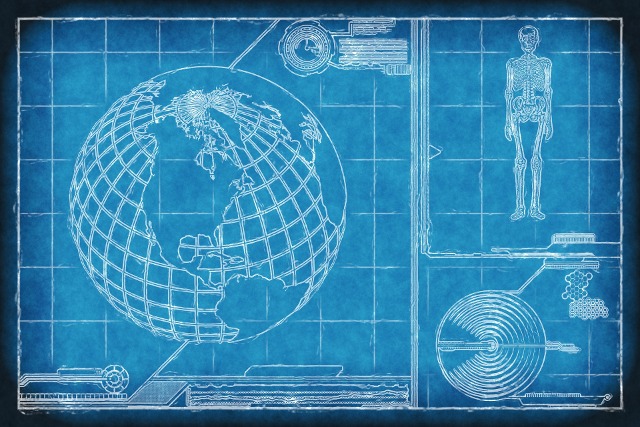
These code examples are intended to help you quickly solve some common everyday tasks in Go. There are also a few oddities that may be nice to have when writing more exotic code.
-
2 basic FIFO queue implementations
Use a slice for a temporary queue. For a long-living queue you should probably use a dynamic data structure, such as a linked list.
-
2 basic set implementations
To implement a set in Go you can use a key-value map with boolean or empty struct values.
-
A basic stack (LIFO) data structure
You can implement a stack (LIFO queue) data structure in Go with a slice and the append function.
-
Access environment variables
Use the Setenv, Getenv, Unsetenv and Environ functions to read and write environment variables.
-
Access private fields with reflection
How to read unexported fields in a struct using reflection in Go.
-
Bitmasks, bitsets and flags
A bitmask or bitset is a set of booleans, often called flags, represented by the bits in one or more numbers number.
-
Check if a number is prime
To check if a number is prime in Go use the ProbablyPrime function from package math/big.
-
Go as a scripting language: lightweight, safe and fast
How to write a basic command-line (CLI) application in Go.
-
Command-line arguments and flags
You can access command-line arguments, including the program name and flags, through the os.Args variable.
-
Compute absolute value of an int/float
Write your own code to compute the absolute value of an integer, but use math.Abs for floating point numbers.
-
Compute max of two ints/floats
Write your own code to compute the minimum and maximum of integers, but use math.Min and math.Max for floating point numbers.
-
Format byte size as kilobytes, megabytes, gigabytes, ...
Utility functions for converting byte size to human-readable format. The code supports both SI and IEC formats.
-
3 simple ways to create an error
How to create simple string-based errors and custom error types with data.
-
Create a new image
To generate a PNG image programmatically in Go use the image, image/color, and image/png packages.
-
Generate all permutations
How to generate all permutations of a slice or string in Go.
-
Hash checksums: MD5, SHA-1, SHA-256
To compute the hash value of a string or byte slice, use the Sum function from crypto package md5, sha1 or sha256. For a file or input stream you need to create a Hash object and write to its Writer function.
-
Hello world HTTP server example
Line-by-line breakdown of a basic HTTP web server with client requests and responses.
-
How to best implement an iterator
Use an iterator function with callbacks to implement a clean and efficient iterator in Go.
-
4 iota enum examples
The iota identifier is used to enumerate constants. For an idiomatic enum implementation, create a new type and give it a String function.
-
Maximum value of an int
The max and min values of an int can be computed as untyped constants.
-
Round float to 2 decimal places
How to round a float to a string/float with 2 decimal places in Go.
-
Round float to integer value
How to round a float64 to the nearest integer: round away from zero, round to even number, convert to an int type.
-
Table-driven unit tests
How to write a table-driven unit test for binary search in Go.
-
The 3 ways to sort in Go
How to sort any type of data in Go using the sort package. All algorithms in the package perform O(n log n) comparisons in the worst case.
Further reading
See the String handling cheat sheet for all about basic string manipulation in Go.