A basic stack (LIFO) data structure
yourbasic.org/golang
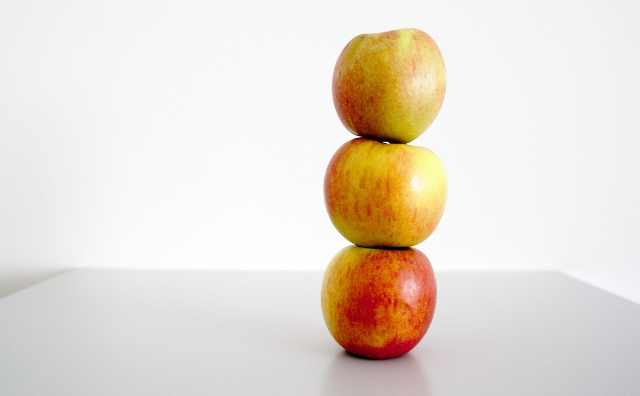
The idiomatic way to implement a stack data structure in Go is to use a slice:
- to push you use the built-in append function, and
- to pop you slice off the top element.
var stack []string
stack = append(stack, "world!") // Push
stack = append(stack, "Hello ")
for len(stack) > 0 {
n := len(stack) - 1 // Top element
fmt.Print(stack[n])
stack = stack[:n] // Pop
}
Hello world!
Performance
Appending a single element to a slice takes constant amortized time. See Amortized time complexity for a detailed explanation.
If the stack is permanent and the elements temporary, you may want to remove the top element before popping the stack to avoid memory leaks.
// Pop
stack[n] = "" // Erase element (write zero value)
stack = stack[:n]
More code examples
Go blueprints: code for common tasks is a collection of handy code examples.