Go go-to guide
yourbasic.org/golang
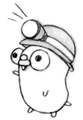
Why Go?
Step-by-step guides
- Go step by step
- Type, value and equality of interfaces
- Create, initialize and compare structs
- Slices/arrays explained: create, index, slice, iterate
- Maps explained: create, add, get, delete
- 5 basic for loop patterns
- 5 switch statement patterns
- Packages explained: declare, import, download, document
Go gotchas
- Assignment to entry in nil map
- Invalid memory address or nil pointer dereference
- Multiple-value in single-value context
- Array won’t change
- See all 27 gotchas
Tutorials
- Go tutorials
- Go beginner’s guide: top 4 resources to get you started
- How to use JSON with Go [best practices]
- Regexp tutorial and cheat sheet
- Java to Go in-depth tutorial
Cheat sheets
- Go string handling overview [cheat sheet]
- Conversions [complete list]
- fmt.Printf formatting tutorial and cheat sheet
- Format a time or date [complete guide]
- Regexp tutorial and cheat sheet
- Bitwise operators [cheat sheet]
- Start a new Go project [standard layout]
- Learn to love your compiler
Code for common tasks
- Go blueprints: code for common tasks
- 2 basic FIFO queue implementations
- 2 basic set implementations
- A basic stack (LIFO) data structure
- Access environment variables
- Access private fields with reflection
- Bitmasks, bitsets and flags
- Check if a number is prime
- Command-line arguments and flags
- Go as a scripting language: lightweight, safe and fast
- Compute absolute value of an int/float
- Compute max of two ints/floats
- 3 simple ways to create an error
- Create a new image
- Format byte size as kilobytes, megabytes, gigabytes, ...
- Generate all permutations
- Hash checksums: MD5, SHA-1, SHA-256
- Hello world HTTP server example
- How to best implement an iterator
- 4 iota enum examples
- Maximum value of an int
- Round float to 2 decimal places
- Round float to integer value
- The 3 ways to sort in Go
- Table-driven unit tests
Concurrent programming
- Concurrent programming
- Goroutines are lightweight threads
- Channels offer synchronized communication
- Select waits on a group of channels
- Data races explained
- How to detect data races
- How to debug deadlocks
- Waiting for goroutines
- Broadcast a signal on a channel
- How to kill a goroutine
- Timer and Ticker: events in the future
- Mutual exclusion lock (mutex)
- 3 rules for efficient parallel computation
Object-oriented programming
- Object-oriented programming without inheritance
- Constructors deconstructed [best practice]
- Methods explained
- Public vs. private
- Pointer vs. value receiver
Functional programming
- Functional programming in Go [case study]
- Function types and values
- Anonymous functions and closures
- How to best implement an iterator
Scripting
StringsCheat sheet
- Go string handling overview [cheat sheet]
- fmt.Printf formatting tutorial and cheat sheet
- Regexp tutorial and cheat sheet
- Runes and character encoding
- Efficient string concatenation [full guide]
- Escapes and multiline strings
- 3 ways to split a string into a slice
- Convert between byte array/slice and string
- Convert between rune array/slice and string
- Convert between float and string
- Convert between int, int64 and string
- Convert interface to string
- Remove all duplicate whitespace
- 3 ways to trim whitespace (or other characters) from a string
- How to reverse a string by byte or rune
MapsStep-by-step
- Maps explained: create, add, get, delete
- 3 ways to find a key in a map
- Get slices of keys and values from a map
- Sort a map by key or value
Slices and arraysStep-by-step
- Slices/arrays explained: create, index, slice, iterate
- 3 ways to compare slices (arrays)
- How to best clear a slice: empty vs. nil
- 2 ways to delete an element from a slice
- Find element in slice/array with linear or binary search
- Last item in a slice/array
Files
- Read a file (stdin) line by line
- Append text to a file
- Find current working directory
- List all files (recursively) in a directory
- Create a temporary file or directory
Time and date
- Format a time or date [complete guide]
- Time zones
- How to get current timestamp
- Get year, month, day from time
- How to find the day of week
- Days between two dates
- Days in a month
- Measure execution time
Random numbers
- Generate random numbers, characters and slice elements
- Generate a random string (password)
- Generate a unique string (UUID, GUID)
- Shuffle a slice or array
- User-friendly access to crypto/rand
Language basics
- Go beginner’s guide: top 4 resources to get you started
- Packages explained: declare, import, download, document
- Package documentation
- Package initialization and program execution order
Statements
- 4 basic if-else statement patterns
- 5 switch statement patterns
- 5 basic for loop patterns
- 2 patterns for a do-while loop in Go
- 4 basic range loop (for-each) patterns
- Defer a function call (with return value)
- Type assertions and type switches
- Type alias explained
Expressions
- Create, initialize and compare structs
- Pointers explained
- Untyped numeric constants with no limits
- Make slices, maps and channels
- How to append anything (element, slice or string) to a slice
- How to use the copy function
- Default zero values for all Go types
- Operators: complete list
- Conversions [complete list]
Methods and interfaces
- Methods explained
- Type, value and equality of interfaces
- Named return values [best practice]
- Optional parameters, default parameter values and method overloading
- Variadic functions (...T)
Error handling
- Error handling best practice
- 3 simple ways to create an error
- Panics, stack traces and how to recover [best practice]
Bits and pieces
- Blank identifier (underscore)
- Find the type of an object
- Generics (alternatives and workarounds)
- Pick the right one: int vs. int64
- 3 dots in 4 places
- Redeclaring variables
Standard library
- How to use JSON with Go [best practices] encoding/json
- fmt.Printf formatting tutorial and cheat sheet fmt
- How to use the io.Reader interface io
- How to use the io.Writer interface io
- Create a new image image
- Write log to file (or /dev/null) log
- Hello world HTTP server example net/http
- Regexp tutorial and cheat sheet regexp
- The 3 ways to sort in Go sort